Local Shared Object (LSO) is the formal name for a "Flash Cookie." For the most part, a Flash Cookie is conceptually the same thing as the better-known HTTP Cookie. The key differences are that Flash Cookies (Local Shared Objects) have a much larger default storage size (100 KB compared to the Internet Cookie's typical 4 KB). Because "Flash Cookies" are so similar to Internet/HTTP cookies, most of the same controversies surrounding cookies apply to both styles equally.
As a web user with a Flash Player installed on your browser, you can control the size of the Flash Cookies (LSOs) stored on your machine for a specific domain. This is easy to do by right-clicking on any Flash-based application in your web browser and selecting the "Settings..." option. The next image is a screen snapshot that shows what appears after selection of "Settings...".
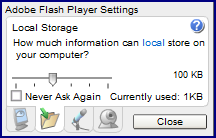
Note in the image above that there are four icons along the bottom of the "Adobe Flash Player Settings" pop-up (next to and left of the "Close" button). The icon that looks like a green arrow pointing into an open folder is the one selected for this screen snapshot shown above. As the snapshot indicates, one can then select the amount of local storage that the domain can use for its Flash Cookies. In this case, the default amount of "100 KB" is still set for the "local" domain (because I ran a Flex-based Flash application hosted on my localhost) and we can see that the cookie is currently 1 KB in size.
So, how do we place data from a Flex-based Flash application into these Flash Cookies and then extract the data back out again? The following code example demonstrates how to do this and the most relevant lines for this discussion are highlighted.
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml"
width="750" height="500">
<mx:Script>
<![CDATA[
/**
* To compile this Flex application from the command line, use
* mxmlc solExample.mxml
*
* This example demonstrates how to use ActionScript to write to, read from,
* and generally manage "Flash cookies" (Local Shared Objects stored on the
* user's host machine to track state for Flash applications).
*/
private const dustinLSO:SharedObject =
SharedObject.getLocal("dustinLocalSharedObject");
/**
* Persist the Local Shared Object (LSO) [AKA Flash Cookie].
*
* @param event Event being handled.
*/
private function persistFlashCookie(event:Event):void
{
// Must access access LSO contents via 'data' property or else see the error
// message "Error: Access of possibly undefined property name through a
// reference with static type flash.net:SharedObject."
dustinLSO.data.name = cookieNameText.text;
dustinLSO.data.text = cookieText.text;
}
/**
* Retrieve and display the Local Shared Object (LSO) contents.
*
* @param event Event being handled.
*/
private function displayFlashCookie(event:Event):void
{
cookieNameDisplay.text = dustinLSO.data.name;
cookieTextDisplay.text = dustinLSO.data.text;
}
]]>
</mx:Script>
<mx:Panel id="mainPanel" title="Showing Off Flash Cookies">
<mx:Form id="mainLsoExampleForm">
<mx:FormItem id="cookieNameItem" label="Name of Flash Cookie">
<mx:TextInput id="cookieNameText" />
</mx:FormItem>
<mx:FormItem id="cookieTextItem"
label="Text to Save to Flash Cookie">
<mx:TextArea id="cookieText" />
</mx:FormItem>
</mx:Form>
<mx:Button label="Persist Flash Cookie" click="persistFlashCookie(event);"/>
<mx:Spacer height="15"/>
<mx:HRule percentWidth="100" strokeWidth="2" strokeColor="blue" horizontalCenter="0" />
<mx:Spacer height="15"/>
<mx:Button label="Retrieve Flash Cookie" click="displayFlashCookie(event);"/>
<mx:Text id="cookieNameDisplay" />
<mx:TextArea id="cookieTextDisplay" />
</mx:Panel>
</mx:Application>
It is difficult in a blog entry to demonstrate persisting of Flash Cookie data that is available between separate executions of the Flash application, but I will attempt to do so with some screen snapshots (click on them to see focused versions) that follow.
The first screen snapshot shows what the Flash application generated from the Flex code above looks like when it first comes up.

The next screen snapshot illustrates placing some text into the name and text fields and pressing the "Persist Flash Cookie" button to write the name and text out to a Flash cookie.

When the "Retrieve Flash Cookie" button is pressed to retrieve data from the local machine stored in the Flash cookie, it is returned as demonstrated in the next screen snapshot.

To make sure that the data is really being stored on the localhost drive rather than simply being in the Flash application's memory, I reloaded the entire application and started by clicking on the "Retrieve Flash Cookie" button. The results are shown in the next screen snapshot.
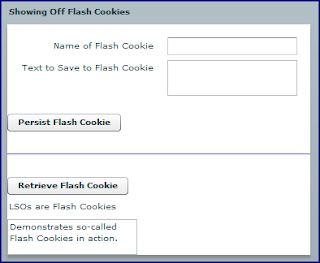
As shown in the last screen snapshot, the data is retrieved from the client machine and so was persisted between completely different executions of the Flash application. In other words, just as traditional Internet Cookies are used for HTTP state management purposes, Flash Cookies similarly support state management between Flash applications execution.
With the overall conceptual description of Flash Cookies covered and a brief introduction to using Flex and Flash Player Action Script class
SharedObject
to read and write Flash Cookies covered, it is time to move on to detecting Flash Cookies on your own machine.Flash applications persist Flash Cookies to a directory that includes a randomly-generated subdirectory name. On the Windows Vista machine I used for the screen snapshots above the Flash Cookies can be found at
C:\Users\Dustin\AppData\Roaming\Macromedia\Flash Player\#SharedObjects\<RANDOM>
. A screen snapshot showing the Windows Explorer perspective on the more specific subdirectory with the Flash Cookie for this entry's Flex example is shown next.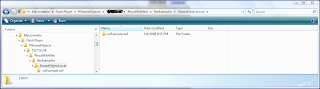
You may be wondering how to best manage/remove Flash Cookies from your own machine. While the Settings option shown at the first of this entry allows you to maintain the size of the cookies, seeing a list of the Flash Cookies on your machine and removing them is most easily done at an Adobe web site specifically designed for this purpose. The Adobe Flash Player Settings Manager is used to manage Flash Cookies. Specifically, the Adobe Flash Player Website Storage Settings Panel at http://www.macromedia.com/support/documentation/en/flashplayer/help/settings_manager07.html allows users to see which Flash Cookies exist on their machine and to manage them.
While Flash Cookies and traditional HTTP Cookies are conceptually similar with similar restrictions on what they can be used for and what they should be used for, there are two major differences. The Flash Cookies (Local Storage Objects) can store significantly more text than the HTTP Cookie counterparts and Flash Cookies require visiting a special web site for removal rather than direct browser removal support (in most cases). A good resource on options for removing and blocking Flash cookies is How Flash Cookies Threaten Your Privacy. It includes a reference to the Firefox extension Objection that is designed to allow for removal of Flash Cookies via Firefox.
When a Flex developer wishes to store data between execution of his or her Flash application on a user's machine, Flash Cookies can be an easy approach for providing a better experience to the end user.
3 comments:
Thanks for writing such a nice article. That was exactly what I was looking for and it works like a charm!
Indigo and Tony,
Thanks for taking the time to let me know this was helpful.
Dustin
still helpful years later ;)
thanks for the detailed step-by-step explanation.
Post a Comment