It is easy to connect to GlassFish via JConsole in Java SE 6 if you are running GlassFish on the same host that you run JConsole on and if the same username is used to start both GlassFish and JConsole. This is easy because JConsole will automatically listed all JMX-exposed MBeans on the same host and running under the same user as the JConsole itself. In fact, JConsole exposes its own MBeans via itself! For this blog entry, however, I wish to focus on remote JMX access of GlassFish and so will demonstrate that next.
When you start GlassFish up with the command
asadmin start-domain
, the console prints out the information you need to access GlassFish's administrative MBeans. The next screen snapshot shows this, including the statement highlighted with a yellow box that says it all: "Standard JMX Clients (like JConsole) can connect to JMXServiceURL: [service:jmx:rmi:///jndi/rmi://MARX-PC:8686/jmxrmi] for domain management purposes."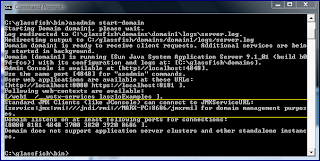
Because GlassFish provides us with the text String for JMXServiceURL, the most difficult part (knowing the JMX Connector URL) is already complete.
If I start up JConsole with the
jconsole
command from the command prompt, I can select the GlassFish MBean Server locally as shown in the next screen snapshot (the highlighted entry "com.sun.enterprise.server.PELaunch start"). This allows me to connect to GlassFish's MBean server without username, password, or URL if I have GlassFish running on the same host and under the same username.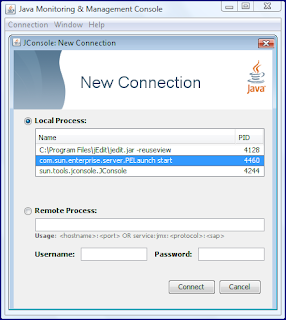
While the above will work for local JMX connections, the remote case is the focus of this entry. I can paste the URL provided by GlassFish (
service:jmx:rmi:///jndi/rmi://MARX-PC:8686/jmxrmi
) in the text field below the "Remote Process" label. This is shown in the next screen snapshot.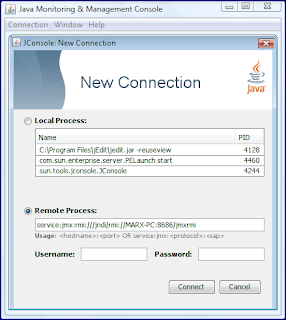
Unfortunately, when I click the "Connect" button with only the remote JMXServiceURL specified without username or password specified, I get the connection error shown in the next screen snapshot:
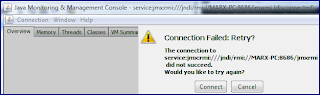
So, GlassFish is expecting credentials (username and password) to be specified. If you use the defaults for GlassFish, these will be a username of
admin
and a password of adminadmin
. Specifying JConsole to connect remotely using these credentials is shown in the next screen snapshot.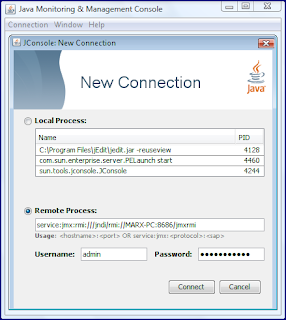
With credentials specified appropriately, JConsole can remotely connect to the running GlassFish JMX MBean server as shown in the next two screen snapshots. The first screen snapshot shows the successful "Connecting ..." message and the second screen snapshot shows a portion of the "MBeans" tab in JConsole.
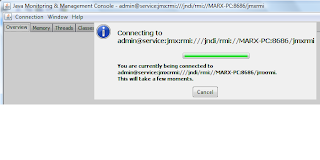
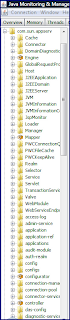
So, we have now been able to connect to GlassFish remotely using JConsole. We can use the same JMXServiceURL and connect to GlassFish from our own JMX clients as well. The remainder of this blog entry demonstrates that.
The following code listing shows a simple JMX client that connects to the same running GlassFish domain instance connected to by JConsole above.
package jmx.dustin;
import java.io.IOException;
import java.net.MalformedURLException;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import javax.management.MBeanServerConnection;
import javax.management.ObjectName;
import javax.management.remote.JMXConnector;
import javax.management.remote.JMXConnectorFactory;
import javax.management.remote.JMXServiceURL;
/**
* Simple example of a JMX client for connecting with GlassFish application
* server.
*/
public class SimpleGlassFishJmxClient
{
/**
* Main executable driver for GlassFish Remote JMX Client. Pass one command
* line parameter to serve as hostname where GlassFish application server
* is running.
*
* @param commandLineArgs Command-line arguments; one expected (host on
* which the GlassFish application server is running).
*/
public static void main(final String[] commandLineArgs)
{
final int port = 8686; // standard GlassFish JMX port.
final String host =
(commandLineArgs.length > 0) ? commandLineArgs[0] : "localhost";
final String jmxGlassFishConnectorString =
"service:jmx:rmi:///jndi/rmi://" + host + ":" + port + "/jmxrmi";
System.out.println( "Using JMXServiceURL string "
+ jmxGlassFishConnectorString);
try
{
final JMXServiceURL jmxUrl =
new JMXServiceURL(jmxGlassFishConnectorString);
final Map jmxEnv = new HashMap();
final String[] credentials = new String[] {"admin", "adminadmin"};
jmxEnv.put( JMXConnector.CREDENTIALS, credentials );
final JMXConnector connector =
JMXConnectorFactory.connect(jmxUrl, jmxEnv);
final MBeanServerConnection mbsc = connector.getMBeanServerConnection();
System.out.println("\nDomains:");
final String domains[] = mbsc.getDomains();
Arrays.sort(domains);
for (String domain : domains)
{
System.out.println("\t" + domain);
}
System.out.println( "\nMBeanServer default domain = "
+ mbsc.getDefaultDomain());
System.out.println("\nMBean count = " + mbsc.getMBeanCount());
System.out.println("\nQuery MBeanServer MBeans:");
@SuppressWarnings("unchecked")
final Set<ObjectName> allObjectNames =
new TreeSet(mbsc.queryNames(null, null));
for (ObjectName objectName : allObjectNames)
{
System.out.println("\t" + objectName);
}
connector.close();
}
catch (MalformedURLException badUrl) // for JMXServiceURL instantiation
{
System.err.println( jmxGlassFishConnectorString
+ " is not a valid JMXServiceURL:\n"
+ badUrl.getMessage() );
}
catch (IOException ioEx) // for JMX/RMI connection
{
System.err.println( "Could not connect to JMX Server using URL "
+ jmxGlassFishConnectorString + ":\n"
+ ioEx.getMessage() );
}
}
}
The code in the example above is adapted from example code available in the highly useful Java Tutorial JMX Trail entry Creating a Custom JMX Client and the Sun Java System Application Server documentation Connecting to the MBean Server entry.
If I had not included the highlighted code above, I would have not been able to connect for the same reason I could not connect with JConsole without specifying a username and password. The next screen snapshot shows the results of trying to connect without username and password.

When I do include username and password as shown in the code above, I achieve success and get the results shown here (WARNING: there is a lot of output here):
Using JMXServiceURL string service:jmx:rmi:///jndi/rmi://localhost:8686/jmxrmi
Domains:
JMImplementation
amx
amx-support
com.sun.appserv
com.sun.jbi
com.sun.jbi.esb
com.sun.management
java.lang
java.util.logging
MBeanServer default domain = DefaultDomain
MBean count = 495
Query MBeanServer MBeans:
JMImplementation:type=MBeanServerDelegate
amx:J2EEApplication=__JWSappclients,J2EEServer=server,WebModule=//server/__JWSappclients,j2eeType=Servlet,name=JWSSystemServlet
amx:J2EEApplication=__JWSappclients,J2EEServer=server,WebModule=//server/__JWSappclients,j2eeType=Servlet,name=default
amx:J2EEApplication=__JWSappclients,J2EEServer=server,WebModule=//server/__JWSappclients,j2eeType=Servlet,name=jsp
amx:J2EEApplication=__JWSappclients,J2EEServer=server,j2eeType=WebModule,name=//server/__JWSappclients
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=DownloadServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=FacesServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=ThemeServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=XmlHttpProxy
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=default
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/,j2eeType=Servlet,name=jsp
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=AdminAPIEntryServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=ConnectServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=RemoteJmxConnectorServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=SynchronizationServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=UploadServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=default
amx:J2EEApplication=null,J2EEServer=server,WebModule=//__asadmin/web1,j2eeType=Servlet,name=jsp
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/,j2eeType=Servlet,name=default
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/,j2eeType=Servlet,name=jsp
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=ActivationCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=ActivationRequesterPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=CompletionCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=CompletionInitiatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=CoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=ParticipantPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=RegistrationCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=RegistrationPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=RegistrationRequesterPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=default
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=Servlet,name=jsp
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=ActivationCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=ActivationRequesterPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=CompletionCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=CompletionInitiatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=CoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=ParticipantPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=RegistrationCoordinatorPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=RegistrationPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/__wstx-services,j2eeType=WebServiceEndpoint,name=RegistrationRequesterPortTypeImpl
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=AuthenticationServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=AxisServlet
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=LPS
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=LZViewer
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=default
amx:J2EEApplication=null,J2EEServer=server,WebModule=//server/laszloExamples,j2eeType=Servlet,name=jsp
amx:J2EEApplication=null,J2EEServer=server,j2eeType=WebModule,name=//__asadmin/
amx:J2EEApplication=null,J2EEServer=server,j2eeType=WebModule,name=//__asadmin/web1
amx:J2EEApplication=null,J2EEServer=server,j2eeType=WebModule,name=//server/
amx:J2EEApplication=null,J2EEServer=server,j2eeType=WebModule,name=//server/__wstx-services
amx:J2EEApplication=null,J2EEServer=server,j2eeType=WebModule,name=//server/laszloExamples
amx:J2EEServer=server,j2eeType=J2EEApplication,name=__JWSappclients
amx:J2EEServer=server,j2eeType=JVM,name=server1211253100850
amx:X-AdminServiceConfig=na,X-ConfigConfig=server-config,X-JMXConnectorConfig=system,j2eeType=X-SSLConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-DASConfig,name=na,X-AdminServiceConfig=na
amx:X-AdminServiceConfig=na,X-ConfigConfig=server-config,j2eeType=X-JMXConnectorConfig,name=system
amx:X-ConfigConfig=server-config,j2eeType=X-EJBTimerServiceConfig,name=na,X-EJBContainerConfig=na
amx:X-ConfigConfig=server-config,X-HTTPListenerConfig=http-listener-2,j2eeType=X-SSLConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-AccessLogConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-ConnectionPoolConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPFileCacheConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPListenerConfig,name=admin-listener,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPListenerConfig,name=http-listener-1,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPListenerConfig,name=http-listener-2,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPProtocolConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-KeepAliveConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-RequestProcessingConfig,name=na,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-VirtualServerConfig,name=__asadmin,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-VirtualServerConfig,name=server,X-HTTPServiceConfig=na
amx:X-ConfigConfig=server-config,X-IIOPListenerConfig=SSL,j2eeType=X-SSLConfig,name=na,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,X-IIOPListenerConfig=SSL_MUTUALAUTH,j2eeType=X-SSLConfig,name=na,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-IIOPListenerConfig,name=SSL,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-IIOPListenerConfig,name=SSL_MUTUALAUTH,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-IIOPListenerConfig,name=orb-listener-1,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-ORBConfig,name=na,X-IIOPServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-JMSHostConfig,name=default_JMS_host,X-JMSServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-ModuleLogLevelsConfig,name=na,X-LogServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=ClientProvider,j2eeType=X-RequestPolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=ClientProvider,j2eeType=X-ResponsePolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=ServerProvider,j2eeType=X-RequestPolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=ServerProvider,j2eeType=X-ResponsePolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=XWS_ClientProvider,j2eeType=X-RequestPolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=XWS_ClientProvider,j2eeType=X-ResponsePolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=XWS_ServerProvider,j2eeType=X-RequestPolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,X-ProviderConfig=XWS_ServerProvider,j2eeType=X-ResponsePolicyConfig,name=na,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,j2eeType=X-ProviderConfig,name=ClientProvider,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,j2eeType=X-ProviderConfig,name=ServerProvider,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,j2eeType=X-ProviderConfig,name=XWS_ClientProvider,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-MessageSecurityConfig=SOAP,j2eeType=X-ProviderConfig,name=XWS_ServerProvider,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-ModuleMonitoringLevelsConfig,name=na,X-MonitoringServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-AuditModuleConfig,name=default,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,X-SecurityServiceConfig=na,j2eeType=X-AuthRealmConfig,name=admin-realm
amx:X-ConfigConfig=server-config,X-SecurityServiceConfig=na,j2eeType=X-AuthRealmConfig,name=certificate
amx:X-ConfigConfig=server-config,X-SecurityServiceConfig=na,j2eeType=X-AuthRealmConfig,name=file
amx:X-ConfigConfig=server-config,j2eeType=X-JACCProviderConfig,name=default,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-MessageSecurityConfig,name=SOAP,X-SecurityServiceConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-ManagerPropertiesConfig,name=na,X-WebContainerConfig=na,X-SessionConfig=na,X-SessionManagerConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-StorePropertiesConfig,name=na,X-WebContainerConfig=na,X-SessionConfig=na,X-SessionManagerConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-SessionManagerConfig,name=na,X-WebContainerConfig=na,X-SessionConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-SessionPropertiesConfig,name=na,X-WebContainerConfig=na,X-SessionConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-SessionConfig,name=na,X-WebContainerConfig=na
amx:X-ConfigConfig=server-config,j2eeType=X-AdminServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-DiagnosticServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-EJBContainerConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-HTTPServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-IIOPServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-JMSServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-JavaConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-LogServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-MDBContainerConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-ManagementRulesConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-MonitoringServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-SecurityServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-ThreadPoolConfig,name=thread-pool-1
amx:X-ConfigConfig=server-config,j2eeType=X-TransactionServiceConfig,name=na
amx:X-ConfigConfig=server-config,j2eeType=X-WebContainerConfig,name=na
amx:X-ServerRootMonitor=server,j2eeType=X-HTTPServiceVirtualServerMonitor,name=server,X-HTTPServiceMonitor=na
amx:X-ServerRootMonitor=server,j2eeType=X-ApplicationMonitor,name=__JWSappclients
amx:j2eeType=X-CallFlowMonitor,name=server,X-ServerRootMonitor=server
amx:X-ServerRootMonitor=server,j2eeType=X-HTTPServiceMonitor,name=na
amx:X-ServerRootMonitor=server,j2eeType=X-JVMMonitor,name=na
amx:j2eeType=X-Logging,name=server,X-ServerRootMonitor=server
amx:X-ServerRootMonitor=server,j2eeType=X-ServerRootMonitor,name=server
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=JBIFramework
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=MEjbApp
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=WSTCPConnectorLCModule
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=WSTXServices
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=__JWSappclients
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=__ejb_container_timer_app
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=adminapp
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=admingui
amx:X-StandaloneServerConfig=server,j2eeType=X-DeployedItemRefConfig,name=laszloExamples
amx:X-StandaloneServerConfig=server,j2eeType=X-ResourceRefConfig,name=jdbc/__CallFlowPool
amx:X-StandaloneServerConfig=server,j2eeType=X-ResourceRefConfig,name=jdbc/__TimerPool
amx:X-StandaloneServerConfig=server,j2eeType=X-ResourceRefConfig,name=jdbc/__default
amx:X-StandaloneServerConfig=server,j2eeType=X-ResourceRefConfig,name=jdbc/sample
amx:j2eeType=J2EEDomain,name=amx
amx:j2eeType=J2EEServer,name=server
amx:j2eeType=X-BulkAccess,name=na
amx:j2eeType=X-ConfigConfig,name=server-config
amx:j2eeType=X-ConfigDottedNames,name=na
amx:j2eeType=X-DeploymentMgr,name=na
amx:j2eeType=X-DomainConfig,name=na
amx:j2eeType=X-DomainRoot,name=amx
amx:j2eeType=X-J2EEApplicationConfig,name=MEjbApp
amx:j2eeType=X-J2EEApplicationConfig,name=__JWSappclients
amx:j2eeType=X-J2EEApplicationConfig,name=__ejb_container_timer_app
amx:j2eeType=X-JDBCConnectionPoolConfig,name=DerbyPool
amx:j2eeType=X-JDBCConnectionPoolConfig,name=SamplePool
amx:j2eeType=X-JDBCConnectionPoolConfig,name=__CallFlowPool
amx:j2eeType=X-JDBCConnectionPoolConfig,name=__TimerPool
amx:j2eeType=X-JDBCResourceConfig,name=jdbc/__CallFlowPool
amx:j2eeType=X-JDBCResourceConfig,name=jdbc/__TimerPool
amx:j2eeType=X-JDBCResourceConfig,name=jdbc/__default
amx:j2eeType=X-JDBCResourceConfig,name=jdbc/sample
amx:j2eeType=X-JMXMonitorMgr,name=na
amx:j2eeType=X-LifecycleModuleConfig,name=JBIFramework
amx:j2eeType=X-LifecycleModuleConfig,name=WSTCPConnectorLCModule
amx:j2eeType=X-MonitoringDottedNames,name=na
amx:j2eeType=X-MonitoringRoot,name=na
amx:j2eeType=X-NotificationEmitterService,name=DomainNotificationEmitterService
amx:j2eeType=X-NotificationServiceMgr,name=na
amx:j2eeType=X-QueryMgr,name=na
amx:j2eeType=X-Sample,name=na
amx:j2eeType=X-StandaloneServerConfig,name=server
amx:j2eeType=X-SystemInfo,name=na
amx:j2eeType=X-UpdateStatus,name=na
amx:j2eeType=X-UploadDownloadMgr,name=na
amx:j2eeType=X-WebModuleConfig,name=WSTXServices
amx:j2eeType=X-WebModuleConfig,name=adminapp
amx:j2eeType=X-WebModuleConfig,name=admingui
amx:j2eeType=X-WebModuleConfig,name=laszloExamples
amx:j2eeType=X-WebServiceMgr,name=na
amx-support:name=amx-debug
amx-support:name=mbean-loader
com.sun.appserv:j2eeType=Servlet,name=JWSSystemServlet,WebModule=//server/__JWSappclients,J2EEApplication=__JWSappclients,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//server/__JWSappclients,J2EEApplication=__JWSappclients,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//server/__JWSappclients,J2EEApplication=__JWSappclients,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//server/__JWSappclients,J2EEApplication=__JWSappclients,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=DownloadServlet,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=FacesServlet,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=ThemeServlet,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=XmlHttpProxy,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=AdminAPIEntryServlet,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=ConnectServlet,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=RemoteJmxConnectorServlet,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=SynchronizationServlet,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=UploadServlet,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//server/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//server/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebServiceEndpoint,name=ActivationCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=ActivationRequesterPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=CompletionCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=CompletionInitiatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=CoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=ParticipantPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=RegistrationCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=RegistrationPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=WebServiceEndpoint,name=RegistrationRequesterPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=Servlet,name=ActivationCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=ActivationRequesterPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=CompletionCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=CompletionInitiatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=CoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=ParticipantPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=RegistrationCoordinatorPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=RegistrationPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=RegistrationRequesterPortTypeImpl,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=AuthenticationServlet,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=AxisServlet,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=LPS,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=LZViewer,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=default,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=Servlet,name=jsp,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//server/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=WebModule,name=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:j2eeType=J2EEApplication,name=__JWSappclients,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=JVM,name=server1211253100850,J2EEServer=server,category=runtime
com.sun.appserv:j2eeType=J2EEDomain,name=com.sun.appserv,category=runtime
com.sun.appserv:j2eeType=J2EEServer,name=server,category=runtime
com.sun.appserv:name=logmanager,category=runtime,server=server
com.sun.appserv:name=domain-status
com.sun.appserv:type=Cache,host=__asadmin,path=/
com.sun.appserv:type=Cache,host=__asadmin,path=/web1
com.sun.appserv:type=Cache,host=server,path=/
com.sun.appserv:type=Cache,host=server,path=/__JWSappclients
com.sun.appserv:type=Cache,host=server,path=/__wstx-services
com.sun.appserv:type=Cache,host=server,path=/laszloExamples
com.sun.appserv:type=Connector,port=4848,address=0.0.0.0
com.sun.appserv:type=Connector,port=8080,address=0.0.0.0
com.sun.appserv:type=Connector,port=8181,address=0.0.0.0
com.sun.appserv:type=DomainDiagnostics,name=server,category=monitor
com.sun.appserv:type=Engine
com.sun.appserv:type=GlobalRequestProcessor,name=http4848
com.sun.appserv:type=GlobalRequestProcessor,name=http8080
com.sun.appserv:type=GlobalRequestProcessor,name=http8181
com.sun.appserv:type=Host,host=__asadmin
com.sun.appserv:type=Host,host=server
com.sun.appserv:type=JVMInformation,category=monitor,server=server
com.sun.appserv:type=JVMInformationCollector,category=monitor,server=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//server/__JWSappclients,J2EEApplication=__JWSappclients,J2EEServer=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//__asadmin/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//__asadmin/web1,J2EEApplication=null,J2EEServer=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//server/,J2EEApplication=null,J2EEServer=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//server/__wstx-services,J2EEApplication=null,J2EEServer=server
com.sun.appserv:type=JspMonitor,name=jsp,WebModule=//server/laszloExamples,J2EEApplication=null,J2EEServer=server
com.sun.appserv:type=Loader,path=/,host=__asadmin
com.sun.appserv:type=Loader,path=/web1,host=__asadmin
com.sun.appserv:type=Loader,path=/,host=server
com.sun.appserv:type=Loader,path=/__JWSappclients,host=server
com.sun.appserv:type=Loader,path=/__wstx-services,host=server
com.sun.appserv:type=Loader,path=/laszloExamples,host=server
com.sun.appserv:type=Manager,path=/,host=__asadmin
com.sun.appserv:type=Manager,path=/web1,host=__asadmin
com.sun.appserv:type=Manager,path=/,host=server
com.sun.appserv:type=Manager,path=/__JWSappclients,host=server
com.sun.appserv:type=Manager,path=/__wstx-services,host=server
com.sun.appserv:type=Manager,path=/laszloExamples,host=server
com.sun.appserv:type=Mapper
com.sun.appserv:type=PWCConnectionQueue,name=http4848
com.sun.appserv:type=PWCConnectionQueue,name=http8080
com.sun.appserv:type=PWCConnectionQueue,name=http8181
com.sun.appserv:type=PWCFileCache,name=http4848
com.sun.appserv:type=PWCFileCache,name=http8080
com.sun.appserv:type=PWCFileCache,name=http8181
com.sun.appserv:type=PWCKeepAlive,name=http4848
com.sun.appserv:type=PWCKeepAlive,name=http8080
com.sun.appserv:type=PWCKeepAlive,name=http8181
com.sun.appserv:type=Realm,path=/,host=__asadmin
com.sun.appserv:type=Realm,path=/web1,host=__asadmin
com.sun.appserv:type=Realm,path=/,host=server
com.sun.appserv:type=Realm,path=/__JWSappclients,host=server
com.sun.appserv:type=Realm,path=/__wstx-services,host=server
com.sun.appserv:type=Realm,path=/laszloExamples,host=server
com.sun.appserv:type=Selector,name=http4848
com.sun.appserv:type=Selector,name=http8080
com.sun.appserv:type=Selector,name=http8181
com.sun.appserv:type=Service,serviceName=null
com.sun.appserv:type=TransactionService,J2EEServer=server,category=runtime
com.sun.appserv:type=Valve,name=BasicAuthenticator,path=/web1,host=__asadmin
com.sun.appserv:type=Valve,name=FormAuthenticator,path=/,host=__asadmin
com.sun.appserv:type=Valve,name=StandardContextValve,path=/,host=__asadmin
com.sun.appserv:type=Valve,name=StandardContextValve,path=/web1,host=__asadmin
com.sun.appserv:type=Valve,name=StandardHostValve,host=__asadmin
com.sun.appserv:type=Valve,name=NonLoginAuthenticator,path=/,host=server
com.sun.appserv:type=Valve,name=NonLoginAuthenticator,path=/__JWSappclients,host=server
com.sun.appserv:type=Valve,name=NonLoginAuthenticator,path=/__wstx-services,host=server
com.sun.appserv:type=Valve,name=NonLoginAuthenticator,path=/laszloExamples,host=server
com.sun.appserv:type=Valve,name=StandardContextValve,path=/,host=server
com.sun.appserv:type=Valve,name=StandardContextValve,path=/__JWSappclients,host=server
com.sun.appserv:type=Valve,name=StandardContextValve,path=/__wstx-services,host=server
com.sun.appserv:type=Valve,name=StandardContextValve,path=/laszloExamples,host=server
com.sun.appserv:type=Valve,name=StandardHostValve,host=server
com.sun.appserv:type=Valve,name=StandardEngineValve
com.sun.appserv:type=access-log,config=server-config,category=config
com.sun.appserv:type=admin-service,config=server-config,category=config
com.sun.appserv:name=__JWSappclients,type=application,category=monitor,server=server
com.sun.appserv:type=application-ref,ref=JBIFramework,server=server,category=config
com.sun.appserv:type=application-ref,ref=MEjbApp,server=server,category=config
com.sun.appserv:type=application-ref,ref=WSTCPConnectorLCModule,server=server,category=config
com.sun.appserv:type=application-ref,ref=WSTXServices,server=server,category=config
com.sun.appserv:type=application-ref,ref=__JWSappclients,server=server,category=config
com.sun.appserv:type=application-ref,ref=__ejb_container_timer_app,server=server,category=config
com.sun.appserv:type=application-ref,ref=adminapp,server=server,category=config
com.sun.appserv:type=application-ref,ref=admingui,server=server,category=config
com.sun.appserv:type=application-ref,ref=laszloExamples,server=server,category=config
com.sun.appserv:type=applications,category=config
com.sun.appserv:type=applications,category=monitor,server=server
com.sun.appserv:type=audit-module,name=default,config=server-config,category=config
com.sun.appserv:type=auth-realm,name=admin-realm,config=server-config,category=config
com.sun.appserv:type=auth-realm,name=certificate,config=server-config,category=config
com.sun.appserv:type=auth-realm,name=file,config=server-config,category=config
com.sun.appserv:type=config,name=server-config,category=config
com.sun.appserv:type=configs,category=config
com.sun.appserv:type=configurator
com.sun.appserv:type=connection-managers,category=monitor,server=server
com.sun.appserv:type=connection-pool,config=server-config,category=config
com.sun.appserv:type=connector-service,category=monitor,server=server
com.sun.appserv:type=controller
com.sun.appserv:type=das-config,config=server-config,category=config
com.sun.appserv:type=diagnostic-service,config=server-config,category=config
com.sun.appserv:type=domain,category=config
com.sun.appserv:name=dotted-name-get-set,type=dotted-name-support
com.sun.appserv:name=dotted-name-monitoring-registry,type=dotted-name-support
com.sun.appserv:name=dotted-name-registry,type=dotted-name-support
com.sun.appserv:type=ejb-container,config=server-config,category=config
com.sun.appserv:type=ejb-timer-service,config=server-config,category=config
com.sun.appserv:type=http-file-cache,config=server-config,category=config
com.sun.appserv:type=http-listener,id=admin-listener,config=server-config,category=config
com.sun.appserv:type=http-listener,id=http-listener-1,config=server-config,category=config
com.sun.appserv:type=http-listener,id=http-listener-2,config=server-config,category=config
com.sun.appserv:type=http-protocol,config=server-config,category=config
com.sun.appserv:type=http-service,config=server-config,category=config
com.sun.appserv:type=http-service,category=monitor,server=server
com.sun.appserv:type=iiop-listener,id=SSL,config=server-config,category=config
com.sun.appserv:type=iiop-listener,id=SSL_MUTUALAUTH,config=server-config,category=config
com.sun.appserv:type=iiop-listener,id=orb-listener-1,config=server-config,category=config
com.sun.appserv:type=iiop-service,config=server-config,category=config
com.sun.appserv:type=j2ee-application,name=MEjbApp,category=config
com.sun.appserv:type=j2ee-application,name=__JWSappclients,category=config
com.sun.appserv:type=j2ee-application,name=__ejb_container_timer_app,category=config
com.sun.appserv:type=jacc-provider,name=default,config=server-config,category=config
com.sun.appserv:type=java-config,config=server-config,category=config
com.sun.appserv:type=jdbc-connection-pool,name=DerbyPool,category=config
com.sun.appserv:type=jdbc-connection-pool,name=SamplePool,category=config
com.sun.appserv:type=jdbc-connection-pool,name=__CallFlowPool,category=config
com.sun.appserv:type=jdbc-connection-pool,name=__TimerPool,category=config
com.sun.appserv:type=jdbc-resource,jndi-name=jdbc/__CallFlowPool,category=config
com.sun.appserv:type=jdbc-resource,jndi-name=jdbc/__TimerPool,category=config
com.sun.appserv:type=jdbc-resource,jndi-name=jdbc/__default,category=config
com.sun.appserv:type=jdbc-resource,jndi-name=jdbc/sample,category=config
com.sun.appserv:type=jms-host,name=default_JMS_host,config=server-config,category=config
com.sun.appserv:type=jms-service,config=server-config,category=config
com.sun.appserv:type=jms-service,category=monitor,server=server
com.sun.appserv:type=jmx-connector,name=system,config=server-config,category=config
com.sun.appserv:type=jndi,category=monitor,server=server
com.sun.appserv:type=jvm,category=monitor,server=server
com.sun.appserv:type=keep-alive,config=server-config,category=config
com.sun.appserv:type=lifecycle-module,name=JBIFramework,category=config
com.sun.appserv:type=lifecycle-module,name=WSTCPConnectorLCModule,category=config
com.sun.appserv:type=log-service,config=server-config,category=config
com.sun.appserv:type=management-rules,config=server-config,category=config
com.sun.appserv:type=manager-properties,config=server-config,category=config
com.sun.appserv:type=mdb-container,config=server-config,category=config
com.sun.appserv:type=message-security-config,name=SOAP,config=server-config,category=config
com.sun.appserv:type=module-log-levels,config=server-config,category=config
com.sun.appserv:type=module-monitoring-levels,config=server-config,category=config
com.sun.appserv:type=monitoring-service,config=server-config,category=config
com.sun.appserv:type=orb,config=server-config,category=config
com.sun.appserv:type=orb,category=monitor,server=server
com.sun.appserv:type=protocolHandler,className=com.sun.enterprise.web.connector.grizzly.GrizzlyHttpProtocol
com.sun.appserv:type=provider-config,name=ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=provider-config,name=ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=provider-config,name=XWS_ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=provider-config,name=XWS_ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=request-policy,provider-config=ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=request-policy,provider-config=ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=request-policy,provider-config=XWS_ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=request-policy,provider-config=XWS_ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=request-processing,config=server-config,category=config
com.sun.appserv:type=resource-ref,ref=jdbc/__CallFlowPool,server=server,category=config
com.sun.appserv:type=resource-ref,ref=jdbc/__TimerPool,server=server,category=config
com.sun.appserv:type=resource-ref,ref=jdbc/__default,server=server,category=config
com.sun.appserv:type=resource-ref,ref=jdbc/sample,server=server,category=config
com.sun.appserv:type=resources,category=config
com.sun.appserv:type=resources,category=monitor,server=server
com.sun.appserv:type=response-policy,provider-config=ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=response-policy,provider-config=ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=response-policy,provider-config=XWS_ClientProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=response-policy,provider-config=XWS_ServerProvider,message-security-config=SOAP,config=server-config,category=config
com.sun.appserv:type=root,category=monitor,server=server
com.sun.appserv:type=security-service,config=server-config,category=config
com.sun.appserv:type=server,name=server,category=config
com.sun.appserv:type=servers,category=config
com.sun.appserv:type=session-config,config=server-config,category=config
com.sun.appserv:type=session-manager,config=server-config,category=config
com.sun.appserv:type=session-properties,config=server-config,category=config
com.sun.appserv:type=ssl,config=server-config,http-listener=http-listener-2,category=config
com.sun.appserv:type=ssl,config=server-config,iiop-listener=SSL,category=config
com.sun.appserv:type=ssl,config=server-config,iiop-listener=SSL_MUTUALAUTH,category=config
com.sun.appserv:type=ssl,config=server-config,jmx-connector=system,category=config
com.sun.appserv:name=//__asadmin/,type=standalone-web-module,category=monitor,server=server
com.sun.appserv:name=//__asadmin/web1,type=standalone-web-module,category=monitor,server=server
com.sun.appserv:name=//server/,type=standalone-web-module,category=monitor,server=server
com.sun.appserv:name=//server/__wstx-services,type=standalone-web-module,category=monitor,server=server
com.sun.appserv:name=//server/laszloExamples,type=standalone-web-module,category=monitor,server=server
com.sun.appserv:type=store-properties,config=server-config,category=config
com.sun.appserv:type=system-services,server=server
com.sun.appserv:type=thread-pool,thread-pool-id=thread-pool-1,config=server-config,category=config
com.sun.appserv:type=thread-pools,config=server-config,category=config
com.sun.appserv:type=thread-pools,category=monitor,server=server
com.sun.appserv:type=transaction-service,config=server-config,category=config
com.sun.appserv:type=transactions-recovery,category=config
com.sun.appserv:type=virtual-server,id=__asadmin,config=server-config,category=config
com.sun.appserv:type=virtual-server,id=server,config=server-config,category=config
com.sun.appserv:name=server,type=virtual-server,category=monitor,server=server
com.sun.appserv:type=web-container,config=server-config,category=config
com.sun.appserv:application=__JWSappclients,name=//server/__JWSappclients,type=web-module,category=monitor,server=server
com.sun.appserv:type=web-module,name=WSTXServices,category=config
com.sun.appserv:type=web-module,name=adminapp,category=config
com.sun.appserv:type=web-module,name=admingui,category=config
com.sun.appserv:type=web-module,name=laszloExamples,category=config
com.sun.jbi:JbiName=server,ServiceName=AdminService,ControlType=AdministrationService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=ConfigurationService,ComponentType=System
com.sun.jbi:JbiName=domain,ServiceName=ConfigurationService,ControlType=DeploymentService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=DeploymentService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=DeploymentService,ControlType=DeploymentService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=AdminService,ControlType=HeartBeat,ComponentType=System
com.sun.jbi:JbiName=domain,ServiceName=ConfigurationService,ControlType=InstallationService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=InstallationService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=InstallationService,ControlType=InstallationService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=AdminService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=DeploymentService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=InstallationService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=LoggingService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=MessageService,ControlType=Lifecycle,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=JBI,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi
com.sun.jbi:JbiName=server,ServiceName=Framework,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.framework
com.sun.jbi:JbiName=server,ServiceName=ManagementService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management
com.sun.jbi:JbiName=server,ServiceName=AdminService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management.AdminService
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management.ConfigurationService
com.sun.jbi:JbiName=server,ServiceName=DeploymentService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management.DeploymentService
com.sun.jbi:JbiName=server,ServiceName=InstallationService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management.InstallationService
com.sun.jbi:JbiName=server,ServiceName=LoggingService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.management.LoggingService
com.sun.jbi:JbiName=server,ServiceName=MessageService,ControlType=Logger,ComponentType=System,LoggerName=com.sun.jbi.messaging
com.sun.jbi:JbiName=domain,ServiceName=ConfigurationService,ControlType=LoggingService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=LoggingService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=LoggingService,ControlType=LoggingService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=MessageService,ControlType=MessageService,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=Framework,ControlType=Statistics,ComponentType=System
com.sun.jbi:JbiName=domain,ServiceName=ConfigurationService,ControlType=System,ComponentType=System
com.sun.jbi:JbiName=server,ServiceName=ConfigurationService,ControlType=System,ComponentType=System
com.sun.jbi:ServiceName=JbiAdminUiService,ComponentType=System
com.sun.jbi:ServiceName=JbiReferenceAdminUiService,ComponentType=System
com.sun.jbi:Target=domain,ServiceName=AdminService,ServiceType=Admin
com.sun.jbi:Target=server,ServiceName=AdminService,ServiceType=Admin
com.sun.jbi:Target=domain,ServiceName=ConfigurationService,ServiceType=Deployment
com.sun.jbi:Target=server,ServiceName=ConfigurationService,ServiceType=Deployment
com.sun.jbi:Target=domain,ServiceName=ConfigurationService,ServiceType=Installation
com.sun.jbi:Target=server,ServiceName=ConfigurationService,ServiceType=Installation
com.sun.jbi:Target=domain,ServiceName=ConfigurationService,ServiceType=System
com.sun.jbi:Target=server,ServiceName=ConfigurationService,ServiceType=System
com.sun.jbi:Target=domain,ServiceName=DeploymentService,ServiceType=Deployment
com.sun.jbi:Target=server,ServiceName=DeploymentService,ServiceType=Deployment
com.sun.jbi:Target=domain,ServiceName=FileTransferService,ServiceType=Download
com.sun.jbi:Target=domain,ServiceName=FileTransferService,ServiceType=Upload
com.sun.jbi:Target=domain,ServiceName=InstallationService,ServiceType=Installation
com.sun.jbi:Target=server,ServiceName=InstallationService,ServiceType=Installation
com.sun.jbi.esb:ServiceType=ArchiveUpload
com.sun.management:type=HotSpotDiagnostic
java.lang:type=ClassLoading
java.lang:type=Compilation
java.lang:type=GarbageCollector,name=Copy
java.lang:type=GarbageCollector,name=MarkSweepCompact
java.lang:type=Memory
java.lang:type=MemoryManager,name=CodeCacheManager
java.lang:type=MemoryPool,name=Code Cache
java.lang:type=MemoryPool,name=Eden Space
java.lang:type=MemoryPool,name=Perm Gen
java.lang:type=MemoryPool,name=Survivor Space
java.lang:type=MemoryPool,name=Tenured Gen
java.lang:type=OperatingSystem
java.lang:type=Runtime
java.lang:type=Threading
java.util.logging:type=Logging
One final thing to note is that any access of a specific MBean associated with GlassFish would be most easily accomplished using client-side proxies as discussed in the "Performing Operations on Remote MBeans via Proxies" section of the previously mentioned Creating Custom JMX Clients tutorial entry and as recommended in the Standard MBean interfaces section of the JMX Best Practices document.
This blog entry has demonstrated how JConsole or a custom Java JMX client can be written to access the MBeans hosted in GlassFish. While GlassFish provides its own excellent web-based administration console that leverages these same MBeans, there may be situations when custom clients need access to the same administrative MBeans.
4 comments:
Excellent!
I had been struggling to get remote JMX working for glassfish for last 3 days. Didn't know that it can be so simle.
Laxmi,
Thanks for taking the time to let me know this was helpful. It's always nice to know when a blog posting has helped someone.
Dustin
Thanks for the information. Do you know where could we find the documentation for the JMX exposed methods for GlassFish? It is kind of useless to have access to the methods if we can not even figure out what do its parameters mean. :(
Xandacona,
The closest thing to this that I am aware of in terms of documentation is the J2EE Management Specification available for download here.
Post a Comment