Code Listing 13 (and Figures 13 and 14) of the OTN article Visualize Your Oracle Database Data with JFreeChart demonstrated how to make a chart's background transparent. In that example, we did not make the plot background transparent because the different color for the plot area often provides a nice offset to the page background. Sometimes, however, you want the entire background, including the plot area, to be transparent. Fortunately, this is very easy to accomplish.
In the following code listing, the method writePngTransparentBasedOnChart()
has been enhanced to make the background of plot area transparent if the last parameter passed to the method is true
.
/** * Write .png file with transparent background based on provided JFreeChart. * * @param aChart JFreeChart. * @param aFileName Name of file to which JFreeChart. * @param aWidth Width of image. * @param aHeight Height of image. * @param aPlotTransparent Should plot area be transparent? */ public void writePngTransparentBasedOnChart( final JFreeChart aChart, final String aFileName, final int aWidth, final int aHeight, final boolean aPlotTransparent ) { final String fileExtension = ".png"; final String writtenFile = destinationDirectory + aFileName + fileExtension; try { aChart.setBackgroundPaint( new Color(255,255,255,0) ); if ( aPlotTransparent ) { final Plot plot = aChart.getPlot(); plot.setBackgroundPaint( new Color(255,255,255,0) ); plot.setBackgroundImageAlpha(0.0f); } final CategoryItemRenderer renderer = aChart.getCategoryPlot().getRenderer(); renderer.setSeriesPaint(0, Color.blue.brighter()); renderer.setSeriesVisible(0, true); // default renderer.setSeriesVisibleInLegend(0, true); // default ChartUtilities.writeChartAsPNG( new FileOutputStream(writtenFile), aChart, aWidth, aHeight, null, true, // encodeAlpha 0 ); System.out.println("Wrote PNG (transparent) file " + writtenFile); } catch (IOException ioEx) { System.err.println( "Error writing PNG file " + writtenFile + ": " + ioEx.getMessage() ); } }
Only a few extra lines were necessary to make the background transparent and these are highlighted. The produced chart with even the plot area transparent is shown in the figure below. This can be contrasted with Figures 13 and 14 in the original article to more easily contrast the difference in the plot area portion of the chart. Click on the image below to see it in larger form.
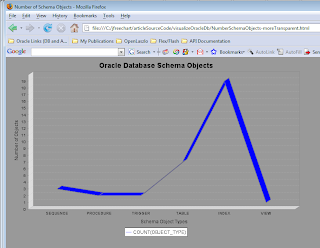
As I stated previously, I often like to have the plot area have a different background color than the page around it for the offset effect, but there are situations where it is nice to have the chart blend more fully into the background page. In such cases, as the listing above demonstrates, it is simple to make the plot areas transparent.
As a reminder, this works because the PNG (Portable Network Graphics) format supports alpha channel transparency.
No comments:
Post a Comment